API Design Best Practices
- Featured Insights
- January 19, 2023
Prefer to use Json format for data exchange:
Use nouns in URL names
GET https://www.example.com/api/v1/employees
POST https://www.example.com/api/v1/employees
PUT https://www.example.com/api/v1/employees/{employee_id}
DELETE https://www.example.com/api/v1/employees/{employee_id}
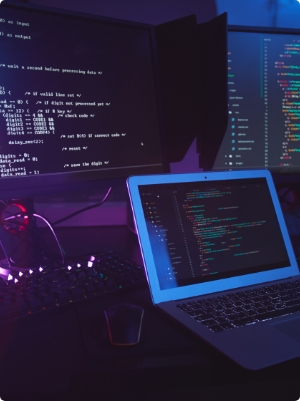
Use the correct http response codes
It is necessary to return a human readable and proper response message when a service is being used. The message must contain the name of the application, a message that concludes on the consumer action, http status code. In case of some error there must be a description of the type of error and a proper error code. Usually, we configure a global error handler in our APIs to handle specific errors.
Errors can occur for various reasons like connection issues from back-end systems, internal errors, expression errors or errors due to passing invalid inputs or data to the API.
There are specific error codes that are defined for each. When so and so error code is displayed, we know where possibly it could have gone wrong. Most popular are http error code and database error code. In mule there is a provision to identify these codes when they occur and deal with them in a specific manner. A proper error response looks something like below.
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- {
- "Success": "false",
- "time": 28-11-2017T11:11:11,
- "title": "employees",
- "errors" : {
- "http_status_code" : 403,
- "message" : "You're not authorized to access this resource."
- "custom_error_code" : 403.1
- }
- }
Use the correct http response codes
Unless you’re creating a publicly available API that does not require users to log in, you must utilize some form of authentication and authorization in your API endpoints.
Authentication proves the identity of an API consumer and verifies usernames, passwords, and access tokens. On the other hand, authorization specifies the different privileges and rights assigned to an end-user.
You should implement a clear policy for granting and denying access to the different resources in your API endpoints. In a more advanced scenario, you might consider using roles and permissions to the group and assign privileges to the API users.
Allow users to decide how much data they want to see
Example:
GET https://www.example.com/api/v1/employees?page=1&per_page=250
Maintain versions for your API
When developing your API, you’ll make changes even after releasing it to the public to improve it and offer more features. You may communicate these changes via email to the end-users, but you must version your API to avoid breaking the client’s applications after each update.
After a new release, always support at least one previous version and deprecate it once you’ve transitioned all consumers. To support this feature, include a version identifier in your API URLs, as shown below.
Example:
https://www.example.com/api/v1
Example:
https://www.example.com/api/v2
Prefer to host your API in the cloud
MuleSoft provides various deployment options, and you need to choose the one that suits your requirement best.
There are Hybrid, on-premises, cloud, rtf deployment options. If you have a choice opt for cloud as it is maintained by MuleSoft and is a false proof environment.
Apply rate limiting policy on your APIs:
To avoid abuse your server resources, rate-limit your API. For example, you can specify the total number of requests that a single user can make in an hour, a day, or a month.
Any account that exceeds that limit should fail with the following HTTP status code. Rate-limiting your API improves its availability and eliminates any chances of Denial-of-Service (DOS) attacks. You can use different technologies to achieve this, including Redis, which works well.
Allow user to sort and filter the data by adding URL and query parameters
Just like sorting data, your API should provide a way to filter data using URL parameters. For instance, to search an item by its name, API consumers can make the following request
DELETE https://www.example.com/api/v1/employees/{employee_id}
Also, sometimes, the end-users may not want to retrieve all the field names. In that case, allow them to specify the columns they want to retrieve using the field parameter as shown below.
GET https://www.example.com/api/v1/products?fields=product_name
Allow your API consumers to specify sorting parameters in the URL to sort data in ascending order. On the other hand, a minus sign – can be prefixed to the field name to sort data in descending order. Here are a few examples of how sorting URLs should look like.
GET https://www.example.com/api/v1/products?sort=product_name